Best of the Basics: JavaScript Informational
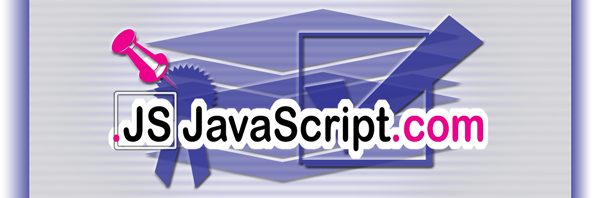
JavaScript Informational: Best of the Basics: A full run through for entry- and mid-level developers looking for some solid fundamentals. Comments and suggestions always welcome.
For those new to the language, this is my own personal introduction to the topic garnered from over 17 years of experience: I’m throwing it out into the informational nexus. -Keith D Commiskey
So you wanna control your browser, ehhh…?
Start with a Solid Base
Variables are the crux of most programming languages, and a good place to start. You have to be able to ask for, handle, save, and recall various tidbits of information.
- Q: Why would you want to create a variable?
- A: Variables are a part of what allows us to control “app”lications—such as your web browser.
Everyone reading this informational on a web browser has the ability to create a variable in less than 30 seconds.
For instance, most browsers allow you to open their “developer tools” using the F12 key.
If you click the “Console” tab at the top of those tools, and type the following at the > prompt:
var myvar = “hello”; and press enter.
For instance, most browsers allow you to open their “developer tools” using the F12 key.
If you click the “Console” tab at the top of those tools, and type the following at the > prompt:
var myvar = “hello”; and press enter.
Congratulations!
Here, myvar is the name of your variable (var is you telling the computer that you are about to create a variable).
Once you assign information to a variable, you can then access that information—by referring to your variable’s name—with other code.
For instance, you can assign numbers to two different variable names, and then add those two variables together.
In doing that, you will have basically just created the ‘addition’ function on a calculator. Bet you didn’t know you’d be doing that today.
Once you assign information to a variable, you can then access that information—by referring to your variable’s name—with other code.
For instance, you can assign numbers to two different variable names, and then add those two variables together.
In doing that, you will have basically just created the ‘addition’ function on a calculator. Bet you didn’t know you’d be doing that today.
- Q: What kind of information can you put in a variable?
- A:
These are called data types, and they refer to things like strings and numbers.
Previously you added a string, “hello”.
Then just now you mentally added a number, which you could do by typing var myvar = 34; and pressing enter.
You can check what data type a variable is holding by using: typeof myvar; which will return either “string” or “number” (or a few others).
In JavaScript, just like string and number, there are seven of these data types, and they’re referred to as “primitive data types”
which just means the base objects themselves cannot be altered (for instance, the number 3 will always be the number 3,
although your myvar reference to the number 3 may change).
which just means the base objects themselves cannot be altered (for instance, the number 3 will always be the number 3,
although your myvar reference to the number 3 may change).
Alongside these seven primitive data types, there is the “object” data type, which is the primary way that we—as developers—work with data.
These objects can also be based on the seven primitive data types, and as a reference (variable assignment) to those primitive data types, their values can be changed.
These objects can also be based on the seven primitive data types, and as a reference (variable assignment) to those primitive data types, their values can be changed.
An Important Note
When assigning a type of data to a variable—be it a string, number,
or other—it should never be changed to a different data type.
If you initially assign var myvar = 3; you should
not try to set it later in the code to be myvar = “3”;
(which is a string—not a number).
or other—it should never be changed to a different data type.
If you initially assign var myvar = 3; you should
not try to set it later in the code to be myvar = “3”;
(which is a string—not a number).
The Base
- Data is one of the underlying fundamentals consistent across every application.
- Variables allow us to handle that data.
- Data Types allow us to keep things organized.
It’s All About the Data
Understanding Data Types
The basis for most things you do with data in JavaScript.
typeof => Data Types => The types of data that are stored in variables.
As your program grows, it will grow harder to keep track of all your variables.
When you get code from other developers, it will grow harder to keep track of all your variables.
With every new feature you add to your web app, it will grow harder to keep track of all your variables.
When you get code from other developers, it will grow harder to keep track of all your variables.
With every new feature you add to your web app, it will grow harder to keep track of all your variables.
- To keep track of your variables, you need to be able to check them.
- You’ll need to see what type of data is in them (if any), or if they even exist (i.e. if they’ve been created).
- Checking data types helps us control the flow of our data.
Important Note: There are two ways a variable comes into being, and we can check for those states.
If the variable hasn’t been created and doesn’t exist at all, it is undeclared (and will throw a Reference error in the browser).
If the variable has been created but hasn’t been assigned anything, it is undefined (which you can—and will—check for regularly).
If the variable hasn’t been created and doesn’t exist at all, it is undeclared (and will throw a Reference error in the browser).
If the variable has been created but hasn’t been assigned anything, it is undefined (which you can—and will—check for regularly).
- Declared: var myVar; // But ‘NOT defined’.
- Defined: myVar = ”; // The = assignment operator will assign a value and define the variable.
This really is important stuff, I promise! If you’re bored … it will pass (or try accounting (j/k!!)). This really is just the basics. You can’t build the house until you learn to swing the hammer (and other cool sayings…) 🙂
And without further ado…
Introducing… Your Data Types!
The typeof operator (or, “command”) will return lowercase strings for each of the data types listed below.
As noted on MDN,
these typeof return strings can be one of the following:
As noted on MDN,
these typeof return strings can be one of the following:
typeof | Result |
---|---|
String | “string“ |
Number | “number“ |
Boolean | “boolean“ |
Undefined | “undefined“ |
Null | “object“ |
Function object | “function“ |
Symbol (new in ECMAScript 2015) | “symbol“ |
BigInt (new in ECMAScript 2020) | “bigint“ |
Any other object | “object“ |
Some Clarity
Number, String, Boolean, Object | ||
|
||
Undefined | ||
|
||
Null | ||
|
||
Symbol, BigInt | ||
|
||
Don’t change data types !!! |
||
|
To the Code!
Comparing … Stuff
Comparators < === > simply compare two data items. For example, 3 == 3 compares two 3s.
The biggest thing here to learn and remember is that there are two ways to compare data items.
-
Compare the Values (between two variables) == and !=
Use 2 character signs to just compare the values.
3 == ‘3’ === true. -
Compare the Values AND the Data Types === and !==
Use 3 character signs to compare the values AND the data types.
3 === ‘3’ === false because one is a number and one is a string. - Best practice is to always use === (especially being that variables should never change what ‘type‘ of values they hold).
Common algebra comparison operators. | < | <= | > | >= |
Check for value only | == | != | ||
Check value and data type | === | !== |
Coding Styles; Standards vs. Preferences
As you learn to code, you will quickly find there are many ways to do things. These ‘options’ can at times lead to industry standards, while others lead more to personal preferences.
Additionally, different industries may have different coding styles.
Additionally, different industries may have different coding styles.
Best practices can at times be subjective; choose your preference.
But! Be able to code in the style of the project you’re working in.
But! Be able to code in the style of the project you’re working in.
Optional: Curly Braces (grouping bits of code)
When using various features of JavaScript, many times you will be provided an option to wrap your code with
{ curly braces }.
When you come across these optional braces… Best practice is to “always” wrap your statements with curly braces.
{ curly braces }.
When you come across these optional braces… Best practice is to “always” wrap your statements with curly braces.
For example, not using them for statements that only have one line (one … “event to run”) is optional.
However, consistency would dictate—because you have to use them when you have two or more lines of code to run—they should always be used.
However, consistency would dictate—because you have to use them when you have two or more lines of code to run—they should always be used.
- Coding Examples:
-
// This will work, and some developers prefer this.
if (alwaysWrapWithBraces === true)
console.log(“One-liners should be wrapped up for consistency.”);
-
// Best practice (subjective)
if (alwaysWrapWithBraces === true) {
console.log(“Consistency is a valued trait in web development.”);
}
Optional: Semicolons
Same holds true for semicolonsat the end of a line;
They are optional, but because there are some gotchas you have to watch out for,
and for which merely introduce more potential entry points for bugs, as with the argument for curly braces, it’s best to just always include them.
and for which merely introduce more potential entry points for bugs, as with the argument for curly braces, it’s best to just always include them.
Parentheses Matter!
In programming, when you need a couple things to happen at the same time—just like with algebra priorities of Addition, Subtration, Multiplication, etc. (PE(MD(AS)))—
there are times when you need to tell the browser in which order things need to be done.
there are times when you need to tell the browser in which order things need to be done.
Such a time in development would be when performing actual math calculations, or sometimes when performing other operations as well.
For these examples, understand that Math.random()
returns a floating-point, pseudo-random number in the range 0–1 (inclusive of 0, but not 1).
Ergo,
returns a floating-point, pseudo-random number in the range 0–1 (inclusive of 0, but not 1).
Ergo,
Math.random() <= 0.999999999999999999 and
Math.random()*2 <= 1.9999999999999999
Math.random()*2 <= 1.9999999999999999
Parentheses Placement | Possible Values |
---|---|
Math.floor(Math.random() * 2 – 1) | // -1 – 0 |
Math.floor(Math.random() * (2 – 1)) | // 1 |
Math.floor(Math.random() * (3 – 1)) | // 0 – 1 |
Math.floor(Math.random() * (3 – 1) + 1) | // 1 – 2 |
Math.floor(Math.random() * 3) | // 0 – 2 |
Your best approach when coding things of this nature is to know what your expected output should be. Then you can perform testing based on your expected output.
For breaking down more complex math problems, you can also try an online calculator such as the “Equation Calculator” I shared in Oct 2019, “Solve for ‘x’ with the Equation Calculator”.
Miscellaneous Tidbits
As you progress in your early learning, the following tidbits of advice should help advance your fundamental understanding of certain things that often go unsaid.
Naming Conventions
-
HTML <tags></tags>: The general concensus is that <html> tags are “almost always” lowercase.
However—technically—it doesn’t matter. But! I look at my code from 2005 and it’s <YELLING AT ME>!
I’ve preferred lowercase ever since that JUMPED out at me one day.
<!DOCTYPE html> is a good exception to this rule. -
HTML ID attributes should simply avoid using kebab-case, and instead use either camelCase or PascalCase.
After many years of seeing differently, this took some major thought adjustment, but my IDs from here-on-out will be PascalCase (aka, TitleCase). - CSS .class-names should be written and named using kebab-case (all-lowercase-with-hyphens).
- JavaScript variable and function names should be written and named using camelCase (not to be confused with TitleCase (or PascalCase)).
Comments are Public
HTML, CSS, and JavaScript code comments can be seen by anyone who can view your web page.
Comments are hidden from the browser’s “viewing” page, but the comments are still accessible if you view the page source (Ctrl|Cmd-U) or explore in your browser’s Developer Tools.
Comments are hidden from the browser’s “viewing” page, but the comments are still accessible if you view the page source (Ctrl|Cmd-U) or explore in your browser’s Developer Tools.
Do NOT put sensitive or similar information in comments.
<u>
The <u> tag is fine to use in some cases;
especially if the site’s <a> link styles are emphasized in some manner
so as not be confused with the standard <u> underline.
Understand the context in which you’re working in.
Be aware of your development environment and a site’s own coding styles.
especially if the site’s <a> link styles are emphasized in some manner
so as not be confused with the standard <u> underline.
Understand the context in which you’re working in.
Be aware of your development environment and a site’s own coding styles.
Copy/Paste
Pasting into Notepad is a nice trick for removing certain hidden characters that hitch a ride when copying sections of data from the internet or various documents (especially with code).
Unfortunately it will not “always” remove all hidden characters.
If problems with a certain section of text in your code persists and is proving challenging,
you can also try pasting into a Word Processor, most of which provide the option to “show hidden characters.”
Unfortunately it will not “always” remove all hidden characters.
If problems with a certain section of text in your code persists and is proving challenging,
you can also try pasting into a Word Processor, most of which provide the option to “show hidden characters.”
A couple approaches I use when I paste things into anywhere—and then begin experiencing issues— (indicating it was due to the paste):
- Delete a chunk of the pasted information, test to see if the issue is still there, then ‘undo’ the delete. Repeat until the error goes away, and narrow it down.
- When you use you left-right arrow keys to scroll through text, you’ll see the cursor scroll happily along.
Hidden characters, however, will cause the cursor to ‘skip a beat’ as it ‘goes over’ the hidden character.
When you see this skip happen, you know there’s something in there, and you can trim it out.
Block vs. Inline vs. Inline-Block Elements
DIVs and SPANs
Understanding “block” vs. “inline” vs. “inline-block” elements is crucial to front-end web development.
MDN has already done an excellent job of explaining these concepts.
Spending one to two days digging deep into these will allow you to better comprehend some even more powerful features
such as Layout mode, Flexbox, and CSS Grid.
such as Layout mode, Flexbox, and CSS Grid.
Padding, Margin
It is extremely crucial to learn these, and no one explains it like MDN!
Border; Outline
According to MDN,
Borders and outlines are very similar. However, outlines differ from borders in the following ways:
- Outlines never take up space, as they are drawn outside of an element’s content.
- According to the spec, outlines don’t have to be rectangular, although they usually are.
- A `border: 5px solid red;` will visibly move/shift adjacent content.
- An `outline: 5px solid red;` is more of an overlay and will not affect the contents.
New HTML Element Types: YMMV (your miles may vary)
Check compatibility charts regularly for target audience browser support.
The MDN docs should be required reading (or at least required skimming).
The MDN docs should be required reading (or at least required skimming).
Every feature has its own chart. MDN especially includes notes on limitations of use, and all the charts usually include mobile browser support.
As you grow with JavaScript, MDN and StackOverflow will typically become your primary go-tos.
Although MDN may appear to be more cryptic early on, you will become more comfortable with it as you progress.
Although MDN may appear to be more cryptic early on, you will become more comfortable with it as you progress.
Tools
- HTML Validation | https://validator.w3.org
- CSS Validation | http://jigsaw.w3.org/css-validator/
Cheat Sheets
- HTML5 cheat sheet | https://websitesetup.org/html5-cheat-sheet/
- Windows keyboard shortcuts | https://www.google.com/search?q=windows+keystrokes
A few common locations for project files
Local: | Location on Computer/Drive | |
Windows | [Desktop, My Documents, etc.] | |
Mac | [Desktop, Documents, etc.] | |
Linux | [/var/www/html/] | |
Remote: | ||
GitHub | Provides free public repositories | |
A “repository” is a virtual folder that stores your project’s files. You can have multiple repositories; each with its own project files. |
||
GitHub Gists | More for code snippets | |
BitBucket | Like GitHub, but isntead of free public repositories, BitBucket provides free private repositories | |
CodePen | Free online text editor with live preview pane | |
Others… |
The #1 essential tip for progressing your skills
Little, Tiny, Side Projects
Familiarity through repetition is a big part of the initial learning curve.
Make yourself a list of “things” you can create… perhaps some that might benefit your own work or study habits.
The more little projects you challenge yourself to do, the more ‘life training badges’ you’ll garner.
The more little projects you challenge yourself to do, the more ‘life training badges’ you’ll garner.
If you can build yourself any little “thing” that does something with HTML, CSS, and JavaScript, you’ll have created a miracle. Build on it from there.
Acronysms
You may come across the acronym a11y; which refers to the word “Accessibility,” which begins with an A, followed by 11 characters and ending in a “y.”
You will also come across this with i18n for internationalization (coding for other languages).
These types of “acronysms” are explained here: https://en.wikipedia.org/wiki/Acronym#Other_conventions
FYI: “acronysms” is a made-up word; although there are 17k Google results. I enjoy research. 😀
Learning Resources
One of the best coding walkthrough and challenge sites for learning.
free Code Camp.org
Good simple, progressive, code exercises
Good simple, progressive, code exercises
Additional Learning Resources.
Good base for code style: W3School JavaScript Tutorial
Deeper base for code style: W3School JavaScript Style Guide and Coding Conventions
Brought to you by
—
Keith D Commiskey
https://keithdc.com
Keith D Commiskey
https://keithdc.com
An additional learning resource for progressing your skills is Flash Cards such as https://ankiweb.net/
AnkiWeb has a tried-and-true algorithm for better brain-burning—it will algorithmically adjust your cards based on your recall level.
An excellent list of suggestions for honing your web development skills (which prompted my addition of flash cards) provided by Tyler S. Lemke @ https://www.linkedin.com/in/tylerslemke/ and can be found on LinkedIn at: https://www.linkedin.com/feed/update/urn:li:activity:6625201378568523776/